# radio组件
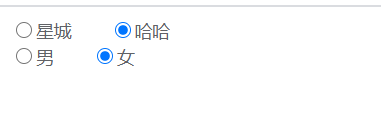
# 前置知识点
radio的基本使用
1
# 参数支持
参数名称 | 参数描述 | 参数类型 | 默认值 |
---|---|---|---|
v-model | 双向绑定 | 布尔类型 | false |
label | 单选框的value值 | string, num, boolean | |
name | name属性 | string |
# 基本结构
- 结构
<label class="xc-radio">
<span class="xc-radio__input">
<span class="xc-radio__inner"></span>
<input type="radio" class="xc-radio__original" />
</span>
<span class="xc-radio__label">我是label</span>
</label>
1
2
3
4
5
6
7
2
3
4
5
6
7
- 样式
.xc-radio{
color: #606266;
font-weight: 500;
line-height: 1;
position: relative;
cursor: pointer;
display: inline-block;
white-space: nowrap;
outline: none;
font-size: 14px;
margin-right: 30px;
-moz-user-select: none;
-webkit-user-select: none;
-moz-user-select: none;
.xc-radio_input{
white-space: nowrap;
cursor: pointer;
outline: none;
display: inline-block;
line-height: 1;
position: relative;
vertical-align: middle;
.xc-radio_inner{
border: 1px solid #dcdfe6;
border-radius: 100%;
width: 14px;
height: 14px;
background-color: #fff;
position: relative;
cursor: pointer;
display: inline-block;
box-sizing: border-box;
&:after{
width: 4px;
height: 4px;
border-radius: 100%;
background-color: #fff;
content: "";
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%,-50%) scale(0);
transition: transform .15s ease-in;
}
}
.xc-radio_original{
opacity: 0;
outline: none;
position: absolute;
z-index: -1;
top: 0;
left: 0px;
right: 0;
bottom: 0;
margin: 0;
}
}
.xc-radio_label{
font-size: 14px;
padding-left: 10px;;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
# 选中的样式
.xc-radio.is-checked {
.xc-radio__input {
.xc-radio__inner {
border-color:#409eff;
background: #409eff;
&:after {
transform: translate(-50%,-50%) scale(1);
}
}
}
.xc-radio__label {
color:#409eff;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
2
3
4
5
6
7
8
9
10
11
12
13
14
# label与插槽的处理
<label class="xc-radio">
<span class="xc-radio__input">
<span class="xc-radio__inner"></span>
<input type="radio" class="xc-radio__original" />
</span>
<span class="xc-radio__label">
<slot></slot>
<!-- 如果没有传内容, 我们就把label当成内容 -->
<template v-if="!$slots.default">{{label}}</template>
</span>
</label>
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# v-model处理
- 接收props数据
props: {
label: {
type: [String, Number, Boolean],
dafault: ""
},
value: null,
name: {
type: String,
default: ""
}
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
- 结构
<input
type="radio"
class="xc-radio__original"
:value="label"
:name="name"
v-model="model"
/>
1
2
3
4
5
6
7
2
3
4
5
6
7
- 提供计算属性
// 需要提供一个计算属性
computed: {
model: {
get() {
return this.value;
},
set(value) {
// 触发父组件给当前组件注册的input事件
this.$emit('input',value)
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12