# 25React原理揭秘
# 1.setState() 的说明
# 1.更新数据
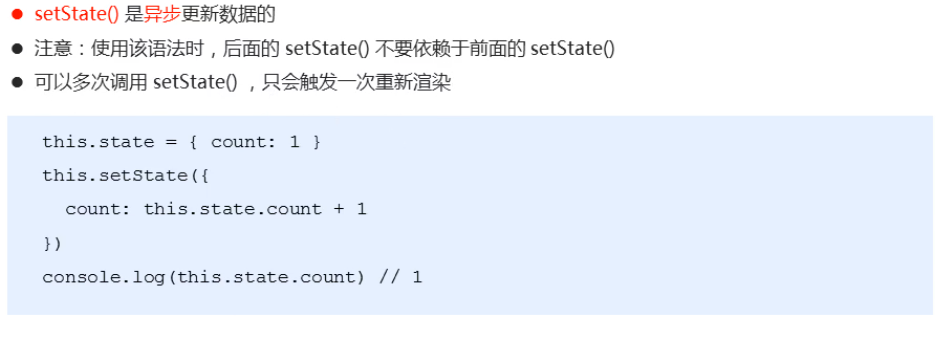
# 2.推荐语法
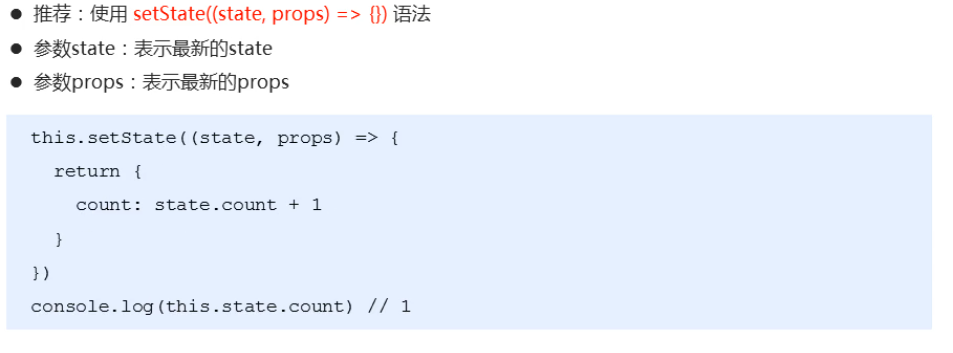
# 3.推荐语法-注意的坑:
# 前置++ 和 后置++ 的问题
// 前置 ++ 先自增再返回, 后置 ++ 先返回,再自增
this.setState((state, props) => {
return {
# 这种后置++是错误的
count: state.count++
# 正确的做法是, 需要使用 前置++
count: ++state.count
}
})
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
# 4.第二个参数
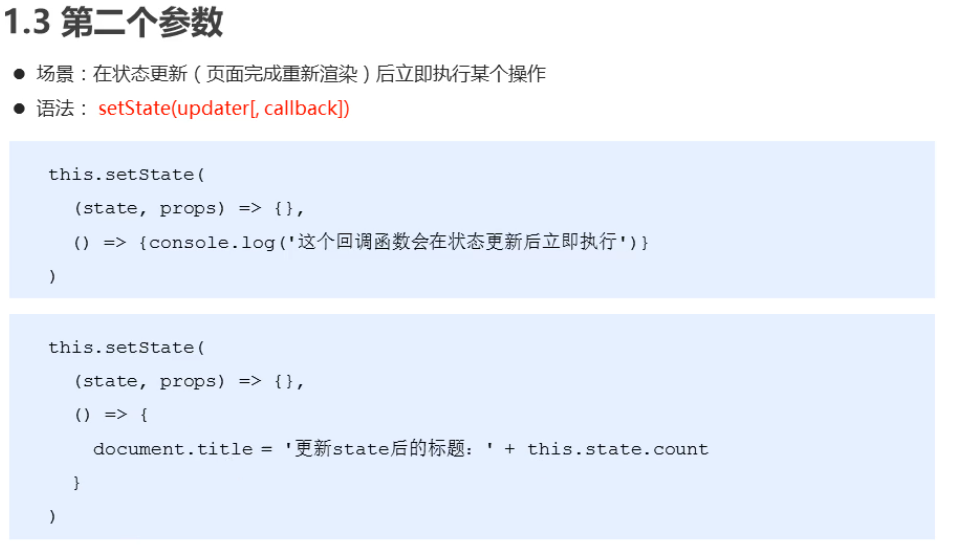
# 2.JSX语法的转化过程
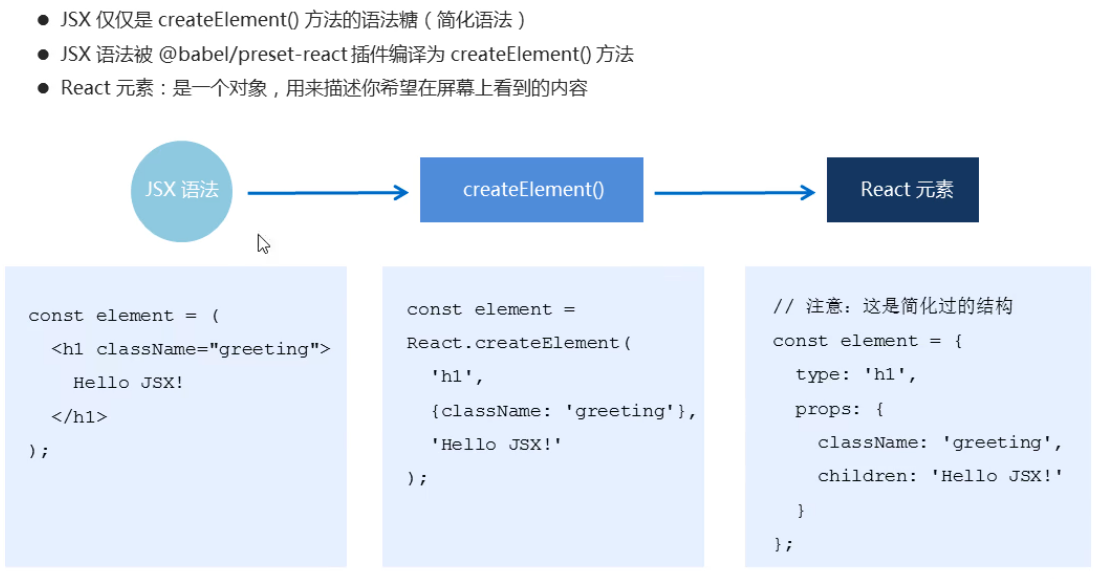
# 3.组件更新机制
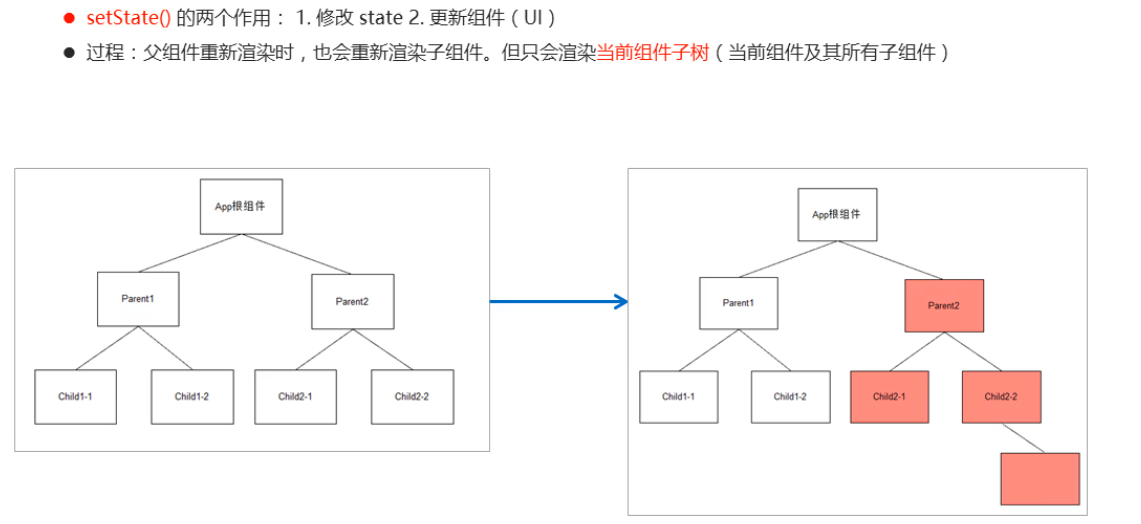
# 4.组件性能优化
# 1.减轻state
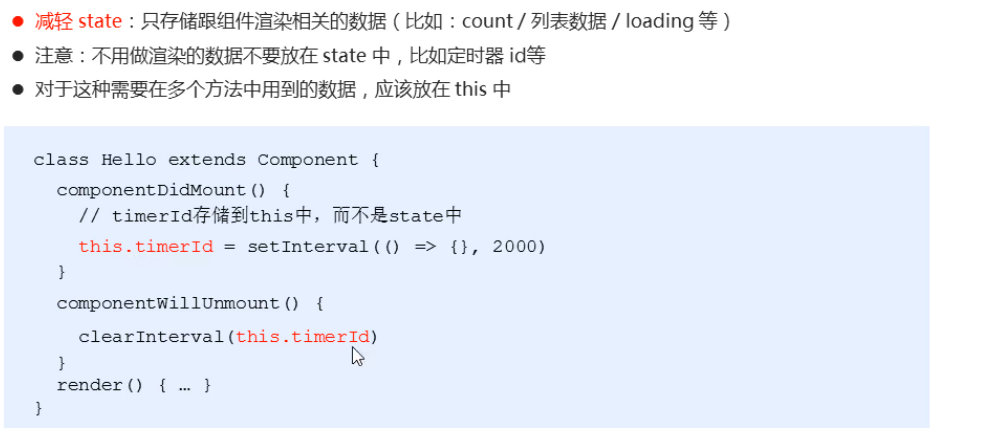
# 2.避免不必要的重新渲染
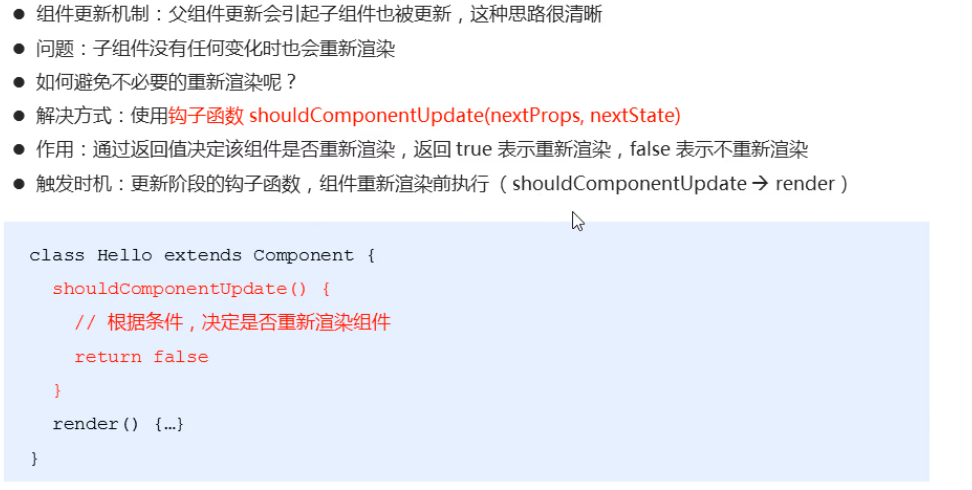
相关代码:
// 钩子函数
shouldComponentUpdate(nextProps, nextState) {
// 返回 false, 阻止组件重新渲染
// return false
// 最新的状态
console.log('最新的state:', nextSate) // 1
// 更新前的状态
console.log('this.state:', this.state) // 0
return true
}
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
# 3.避免不必要的重新渲染 - 随机数案例1
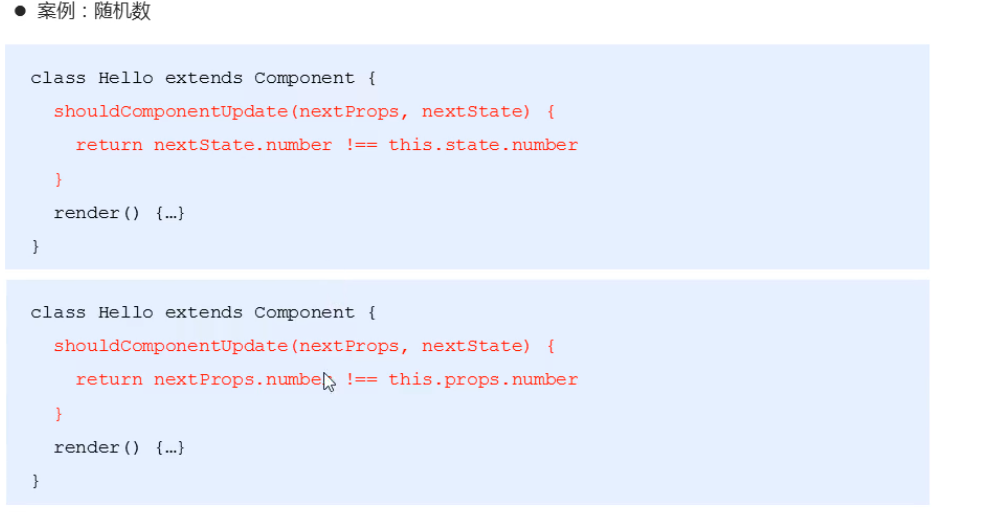
相关代码:
class Random extends React.Component {
state = {
number: 0
}
handleClick = () => {
this.setState(() => {
return {
number: Math.floor(Math.random() * 3)
}
})
}
shouldComponentUpdate(nextProps, nextSate) { ⭐⭐⭐
console.log('最新的状态: ',nextSate ,'当前状态: ',this.state)
if(nextSate.number === this.state.number) {
return false
}
return true
}
render() {
console.log('render')
return (
<div>
<h1>随机数: {this.state.number}</h1>
<button onClick={this.handleClick}>重新生成</button>
</div>
)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
# 4.避免不必要的重新渲染 - 随机数案例2
class Random extends React.Component {
state = {
number: 0
}
handleClick = () => {
this.setState(() => {
return {
number: Math.floor(Math.random() * 3)
}
})
}
render() {
return (
<div>
<NumberBox number={this.state.number}/>
<button onClick={this.handleClick}>重新生成</button>
</div>
)
}
}
class NumberBox extends React.Component { ⭐⭐⭐
shouldComponentUpdate(nextProps) {
console.log('最新的props: ',nextProps,'当前props: ',this.props)
// 如果前后两次的number值相同, 就返回false, 不更新组件
if(nextProps.number === this.props.number) {
return false
}
return true
}
render() {
console.log('子组件中的render')
return <h1>随机数: {this.props.number}</h1>
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
# 5.纯组件 - 基本使用
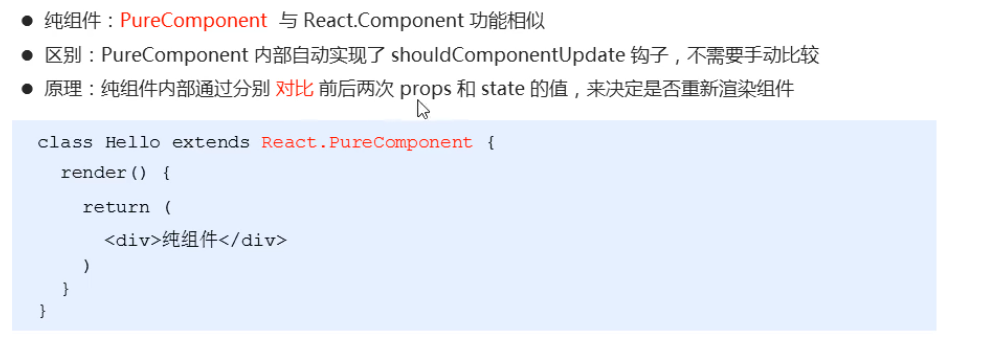
# 6.纯组件-shallow compare
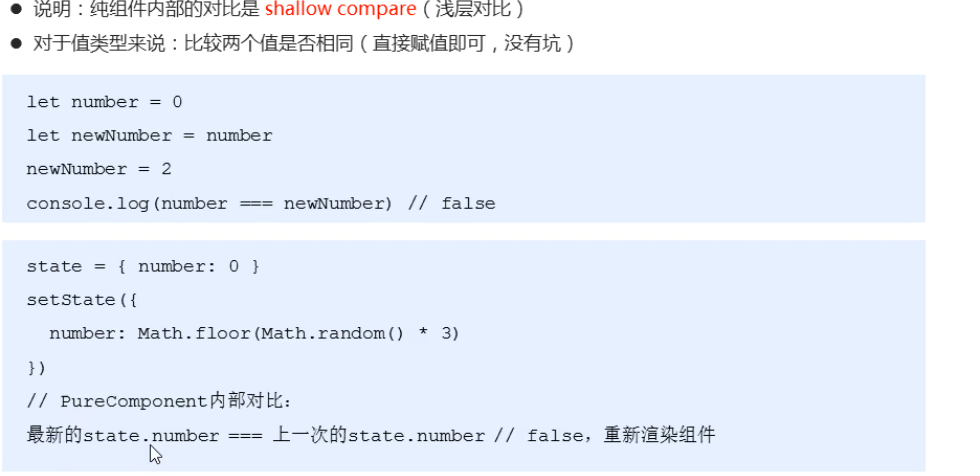
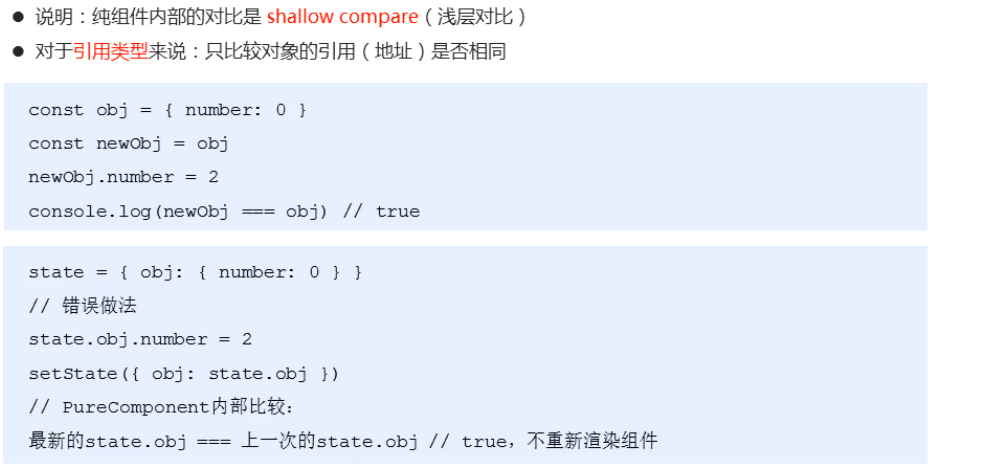
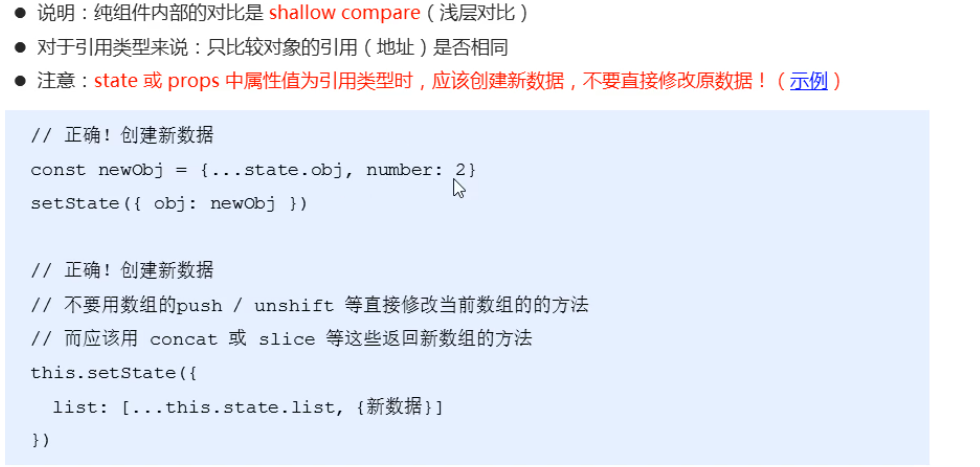
# 5.虚拟DOM 和 Diff 算法

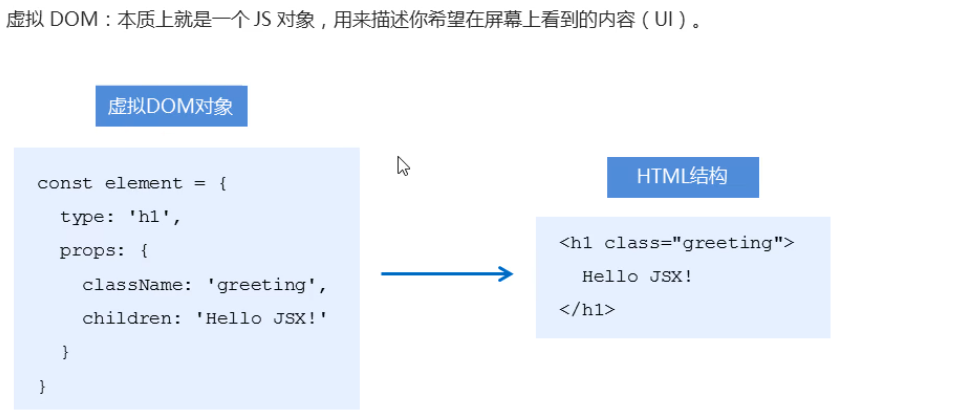
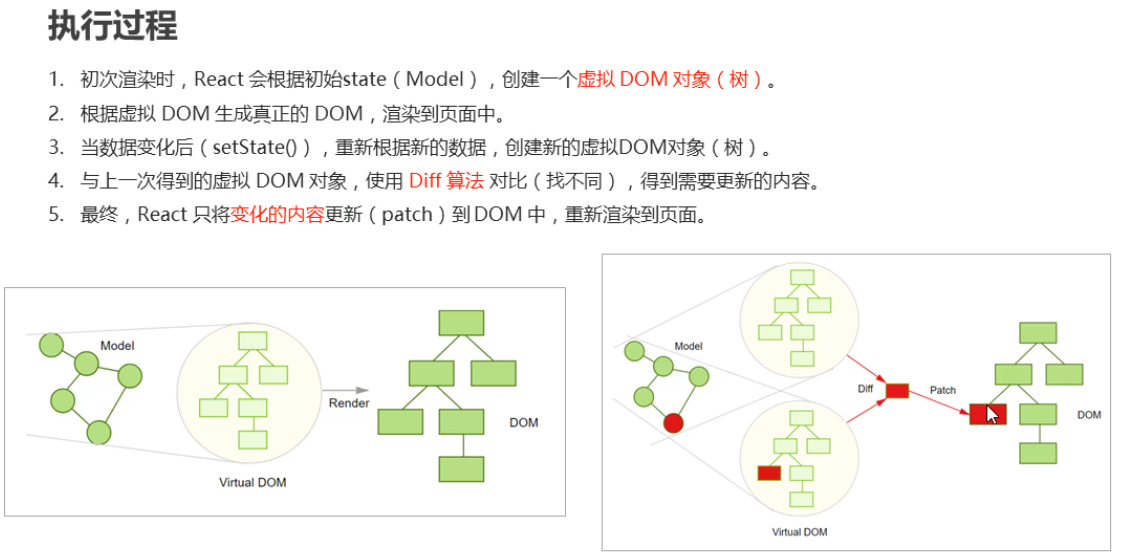
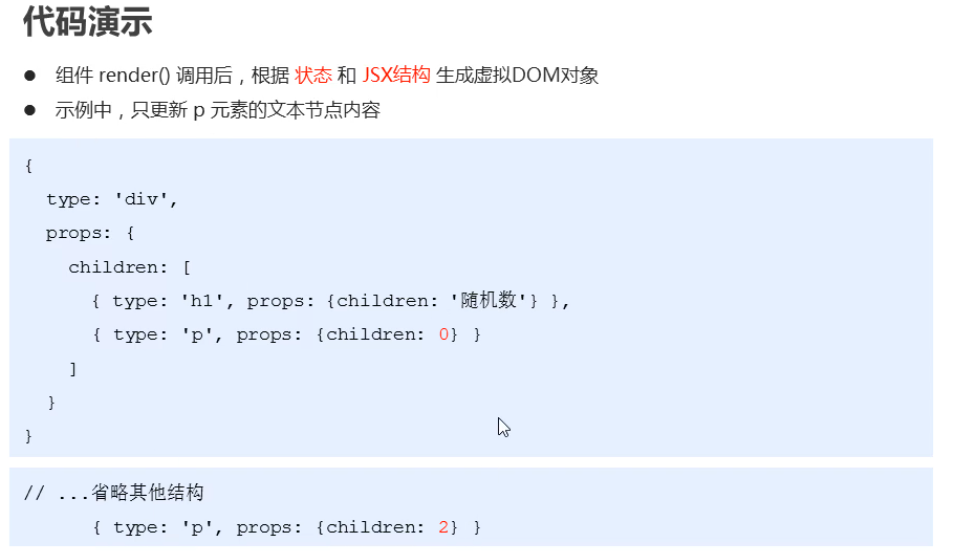
# 6.React原理揭秘总结
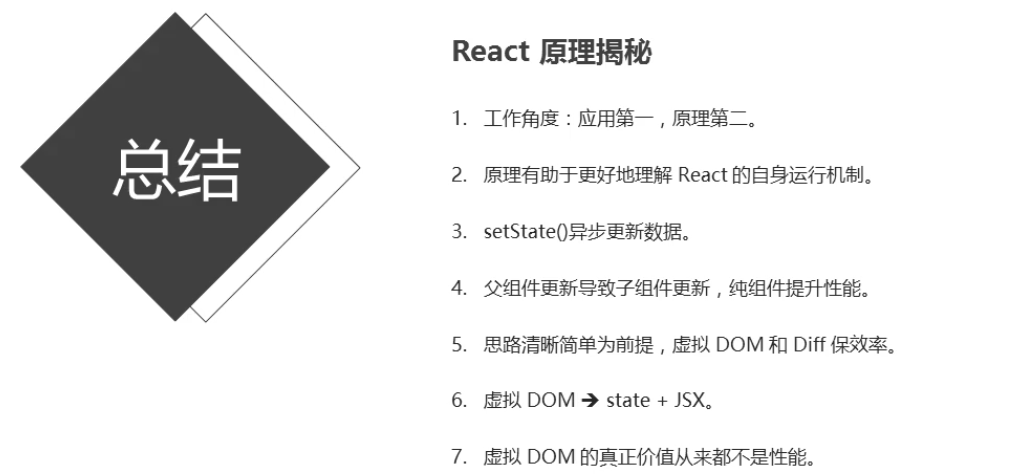