# 18.多媒体接口, 实现自定义播放器
# 1.常用方法:
load() 加载 play() 播放 pause() 暂停
Jq没有提供对视频播放控件的方式, 也就意味着如果要操作视频播放, 必须使用原生的js方法-dom元素
# 2.常用属性:
a) currentTime 视频播放的当前进度
b) duration: 视频的总时间 100000/60
c) paused: 视频播放的状态
# 3.常用事件:
a) oncanplay: 事件在用户可以开始播放视频/音频(auto/video)时触发
b) ontimeupdate: 通过该事件来报告当前的播放进度
c) onended: 播放完时触发-重置
# 视频播放器效果
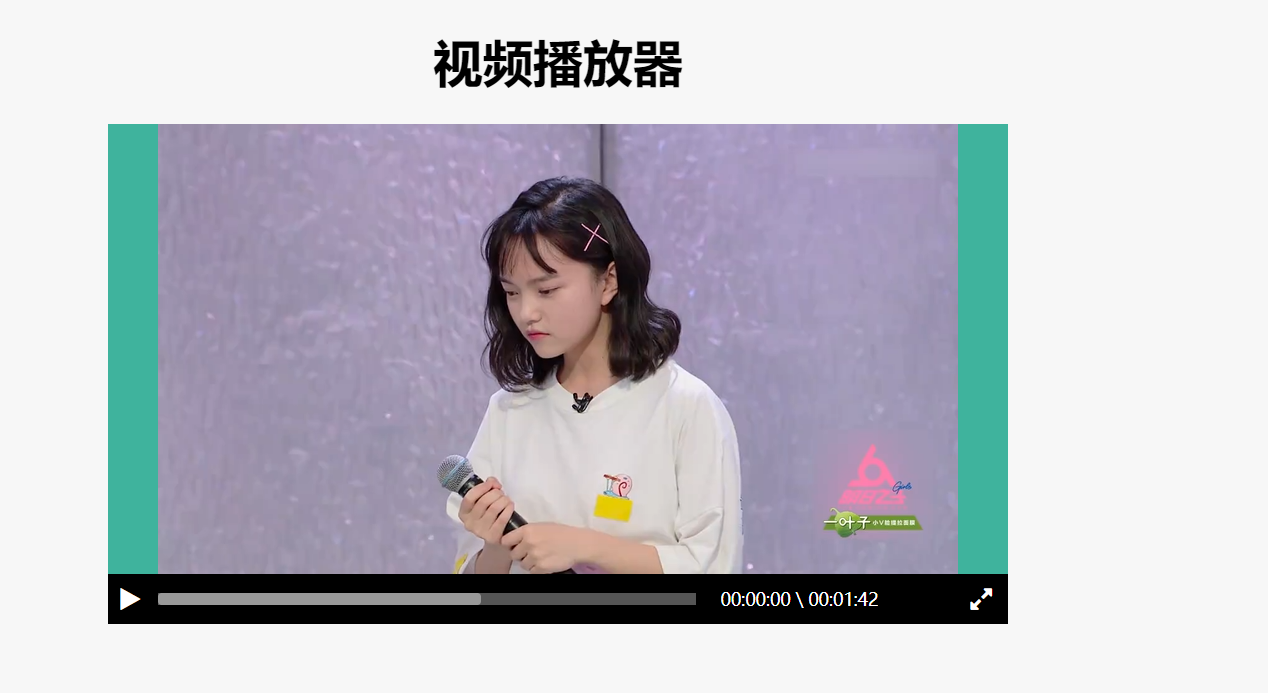
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<link rel="stylesheet" href="css/font-awesome.css">
<link rel="stylesheet" href="css/font-awesome.min.css">
<link rel="stylesheet" href="css/css.css">
</head>
<body>
<h3 class="playerTitle">视频播放器</h3>
<div class="player">
<video src="audio/xc.mp4"></video>
<div class="controls">
<a href="javascript:;" class="switch fa fa-play"></a> <!-- 暂停播放切换按钮 -->
<a href="javascript:;" class="expand fa fa-expand"></a> <!-- 全屏按钮 -->
<div class="progress"> <!-- 进度条 -->
<div class="bar"></div>
<div class="loaded"></div>
<div class="elapse"></div>
</div>
<div class="time">
<span class="currentTime">00:00:00</span>
\
<span class="totalTime">00:00:00</span>
</div>
</div>
</div>
<script src="js/jquery-1.12.4.min.js"></script>
<script>
// 通过jq来实现功能
$(function(){
// 1.获取播放器
var video=$("video")[0];
// 2.实现播放和暂停
$(".switch").click(function(){
// 实现播放和暂停的切换: 如果是暂停 》》 播放 如果是播放 》》 暂停
if(video.paused){
video.play();
// 移除暂停的样式,添加播放样式
}
else{
video.pause();
// 移除播放样式,添加暂停样式
}
// 设置标签的样式 fa-play:播放的样式 fa-pause: 暂停的样式
$(this).toggleClass("fa-play fa-pause");
});
// 3.实现全屏操作
$(".expand").click(function(){
// 全屏 》》 不同浏览器需要添加不同前缀 》》 能力测试
if(video.requestFullScreen){
video.requestFullScreen();
}
else if(video.webkitRequestFullScreen){
video.webkitRequestFullScreen();
}
else if(video.mozRequestFullScreen){
video.mozRequestFullScreen();
}
else if(video.msRequestFullScreen){
video.msRequestFullScreen();
}
});
// 4.实现播放的业务逻辑: 当视频文件可以播放时触发下面的事件
video.oncanplay=function(){
// 1.将视频文件设置为显示
setTimeout(function(){
video.style.display="block";
// 2.获取当前视频文件的总时长(有小数值), 计算时分秒
var total=video.duration;
// 3.计算时分秒 60*60=3600
// var hour = Math.floor(total/3600);
// 补0操作
// hour = hour<10?"0"+hour:hour;
// var minute = Math.floor(total%3600/60);
// minute = minute<10?"0"+minute:minute;
// var second = Math.floor(total%60);
// second = second<10?"0"+second:second;
var result = getResult(total)
// 4.将计算的结果展示在dom元素中
$(".totalTime").html(result)
},300);
}
// 通过总时长计算出时分秒
function getResult(time){
var hour = Math.floor(time/3600);
// 补0操作
hour = hour<10?"0"+hour:hour;
var minute = Math.floor(time%3600/60);
minute = minute<10?"0"+minute:minute;
var second = Math.floor(time%60);
second = second<10?"0"+second:second;
// 返回结果
return hour+":"+minute+":"+second
}
// 5.实现播放过程中的业务逻辑,当视频播放时会触发ontimeupdate事件
// 如果修改currentTime值也会触发这个事件
video.ontimeupdate=function(){
// 1.获取当前的播放时间
var current = video.currentTime;
// 2.计算时分秒
var result = getResult(current);
// 3.将结果展示在指定的DOM元素中
$(".currentTime").html(result);
// 4.设置当前进度条样式 0.12 >> 0.12*100+"%" >> 12%
var percent = current/video.duration*100+"%";
$(".elapse").css("width", percent);
}
// 6.实现视频的跳播
$(".bar").click(function(e){
console.log(e)
// 1.获取当前鼠标相对于父元素的left值 -- 偏移值
var offset = e.offsetX;
// 2.计算偏移值相对于总父元素总宽度的比例
var percent = offset/$(this).width();
// 3.计算这个位置对应的视频进度值
var current = percent*video.duration;
// 4.设置当前视频的currentTime
video.currentTime=current;
});
// 7.播放完毕之后, 重置播放器的状态
video.onended=function(){
video.currentTime=0;
$(".switch").removeClass("fa-pause").addClass("fa-play");
}
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137