# 5.简约之美AVA
简单的说ava是mocha的替代品:
- es6语法支持更好,对aysnc/await有支持
- 执行效率更高,使用io并发,就必须保证测试的原子性
- 语义上更简单,集众家之长
虽然 JavaScript 是单线程,但在 Node.js 里由于其异步的特性使得 IO 可以并行。AVA 利用这个优点让你的测试可以并发执行,这对于 IO 繁重的测试特别有用。另外,测试文件可以在不同的进程里并行运行,让每一个测试文件可以获得更好的性能和独立的环境。
# AVA特点
轻量和高效
简单的测试语法
并发运行测试
强制编写原子测试
一旦开始,就一直运行到结束,中间不会切换到另一个测试
没有隐藏的全局变量
为每个测试文件隔离环境
用 ES2015 编写测试
支持 Promise
支持 Generator
支持 Async
支持 Observable
强化断言信息
可选的 TAP 输出显示
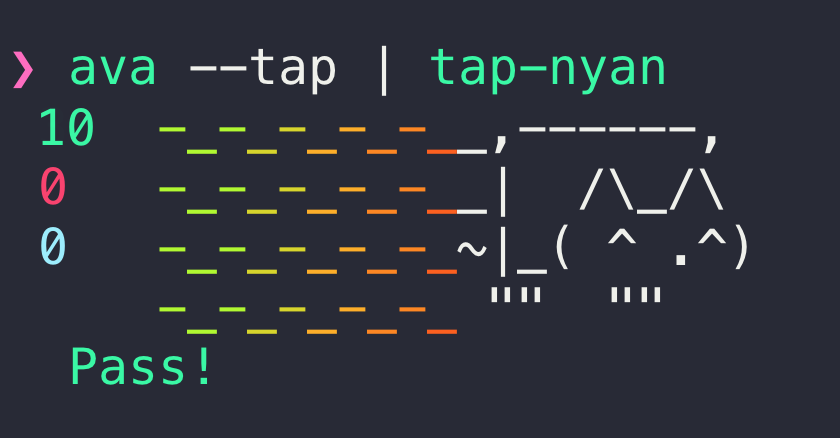
- 简明的堆栈跟踪
# 安装&开始
情景一:
// 创建一个ava项目
npm init ava
2
形成package.json
{
"name": "awesome-package",
"scripts": {
"test": "ava"
},
"devDependencies": {
"ava": "^1.0.0"
}
}
2
3
4
5
6
7
8
9
情景二:(推荐)
npm init -y
// npm & cnpm
npm install -D ava
// yarn
yarn add ava -D
2
3
4
5
6
7
测试ava正常安装:
➜ npx ava --version
2.2.0
2
# 第一个ava测试例子
流程:
- 引用ava的测试API
- 执行测试
- 使用断言
创建test.js
文件
import test from 'ava';
const testfn = (a, b) => a + b
test('hello ava', t => {
t.pass();
});
test('my first test', async t => {
const str = 'hello ava!!!!'
t.is(str, 'hello ava!!!!')
});
test('add method', async t => {
const result = testfn(3, 4)
t.is(result, 7)
});
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
ava自动搜索如下文件结尾的文件:
**/test.js
**/test-*.js
**/*.spec.js
**/*.test.js
**/test/**/*.js
**/tests/**/*.js
**/__tests__/**/*.js
ava中的断言:
.pass([message])
.fail([message])
.assert(value, [message])
.truthy(value, [message])
.falsy(value, [message])
.true(value, [message])
.false(value, [message])
.is(value, expected, [message])
.not(value, expected, [message])
.deepEqual(value, expected, [message])
.notDeepEqual(value, expected, [message])
.deepEqual()。
.throws(fn, [expected, [message]])
.throwsAsync(thrower, [expected, [message]])
.notThrows(fn, [message])
.notThrowsAsync(nonThrower, [message])
.regex(contents, regex, [message])
.notRegex(contents, regex, [message])
.snapshot(expected, [message])
.snapshot(expected, [options], [message])
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
# CLI命令
使用--help
命令去查看ava支持的cli参数
➜ npx ava --help
Testing can be a drag. AVA helps you get it done.
Usage
ava [<file> ...]
Options
--watch, -w Re-run tests when tests and source files change
--match, -m Only run tests with matching title (Can be repeated)
--update-snapshots, -u Update snapshots
--fail-fast Stop after first test failure
--timeout, -T Set global timeout (milliseconds or human-readable, e.g. 10s, 2m)
--serial, -s Run tests serially
--concurrency, -c Max number of test files running at the same time (Default: CPU cores)
--verbose, -v Enable verbose output
--tap, -t Generate TAP output
--color Force color output
--no-color Disable color output
--reset-cache Reset AVA's compilation cache and exit
--config JavaScript file for AVA to read its config from, instead of using package.json
or ava.config.js files
Examples
ava
ava test.js test2.js
ava test-*.js
ava test
The above relies on your shell expanding the glob patterns.
Without arguments, AVA uses the following patterns:
**/test.js **/test-*.js **/*.spec.js **/*.test.js **/test/**/*.js **/tests/**/*.js **/__tests__/**/*.js
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
# 文件匹配
使用match
指令,匹配对应需要测试的文件:
匹配标题以foo
:结尾
npx ava --match ='* foo'
匹配标题以foo
:
npx ava --match ='foo *'
匹配标题包含foo
:
npx ava --match ='* foo *'
匹配是完全相同 foo
:
npx ava --match ='foo'
匹配标题不包含foo
:
npx ava --match ='!* foo *'
匹配以下foo
结尾的标题bar
:
npx ava --match ='foo * bar'
匹配foo
以bar
:开头或结尾的标题:
npx ava --match ='foo *' - match ='* bar'
# 关于reporter
默认情况下,AVA使用最小的报告:

使用该--verbose
标志启用详细的报告者。除非启用TAP报告,否则始终在CI环境中使用此选项。
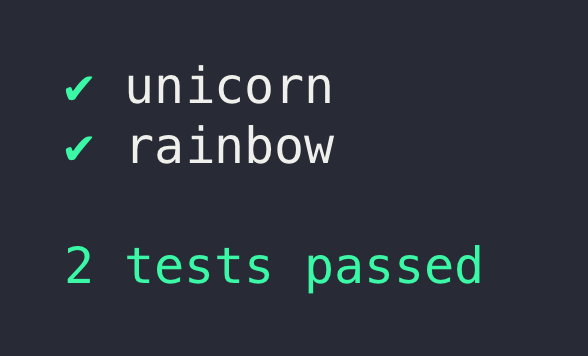
TAP报告(推荐)
AVA支持TAP格式,因此与任何TAP报告器兼容。使用该--tap
标志启用TAP输出。
$ npx ava --tap | npx tap-nyan
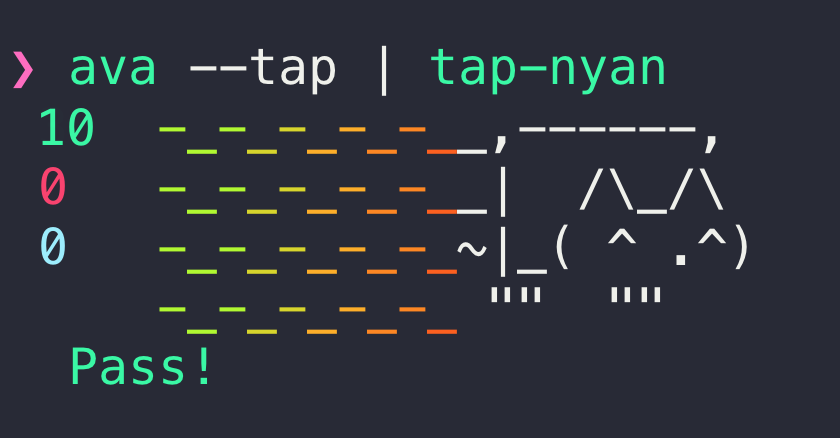
这里有一些格式:
- tap-dot (opens new window) - Dotted output.
- tap-spec (opens new window) - Mocha-like spec reporter.
- tap-nyan (opens new window) - Nyan cat.
- tap-min (opens new window) - Minimal output.
- tap-difflet (opens new window) - Minimal output with diffing.
- tap-diff (opens new window) - Human-friendly output with diffing.
- tap-simple (opens new window) - Simple output.
- faucet (opens new window) - Human-readable summarizer.
- tap-mocha-reporter (opens new window) - Use any of the Mocha reporters (opens new window).
- tap-summary (opens new window) - Summarized output.
- tap-pessimist (opens new window) - Only shows failed tests.
- tap-prettify (opens new window) - Nice readable output with diffing.
- tap-colorize (opens new window) - Colorize the output while preserving machine-readability.
- tap-bail (opens new window) - Bail out when the first test fails.
- tap-notify (opens new window) - Notifier for macOS, Linux and Windows.
- tap-json (opens new window) - JSON output.
- ava-tap-json (opens new window) - JSON output with AVA compatibility.
- tap-xunit (opens new window) - xUnit output.
- tap-teamcity (opens new window) - Output for TeamCity.
# 快照功能
ava自动进行项目测试快照,如果文件放置在test
或者tests
目录,则快照会放置在snapshots
目录。如果测试放置在__test__
目录,则快照放置在__snapshots__
目录
ava --update-snapshots
可以指定一个固定位置,以便在AVA的package.json
配置中存储快照文件:
package.json:
{
"ava":{
"snapshotDir":"自定义目录"
}
}
2
3
4
5
# 设置超时
AVA中的超时行为与其他测试框架中的行为不同。AVA在每次测试后重置计时器,如果在指定的超时内没有收到新的测试结果,则强制测试退出。这可用于处理停滞的测试。
没有默认超时。
您可以配置使用超时--timeout
命令行选项,或配置文件中设置。它们可以以人类可读的方式设置:
# 10秒
npx ava --timeout = 10s
# 2分钟
npx ava --timeout = 2m
# 100毫秒
npx ava --timeout = 100
2
3
4
5
6
7
8
还可以为每个测试单独设置超时。每次进行断言时都会重置这些超时。
test('foo', t => {
t.timeout(100); // 100 milliseconds
// Write your assertions here
});
2
3
4
# 其他ava设置相关
# ESLint
如果使用了ESLint,请添加eslint-plugin-ava (opens new window)
{
plugins: [
"ava"
]
}
2
3
4
5
# 异步相关
如果异步操作使用promises,则应返回promise:
test('fetches foo', t => {
return fetch().then(data => {
t.is(data, 'foo');
});
});
2
3
4
5
更好的是,使用async
/ await
:
test('fetches foo', async t => {
const data = await fetch();
t.is(data, 'foo');
});
2
3
4