# WebSocket服务端
常用的 Node 实现有以下几种。
- ws (opens new window)
- µWebSockets (opens new window)
- Socket.IO (opens new window)
- WebSocket-Node (opens new window)
# ws配置服务端
npm install -S ws
1
使用Wesocket.Server
方法,可以快速初始化服务端:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
console.log('received: %s', message);
});
ws.send('something');
});
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
客户端的例子:
// 引用weboscket库
const WebSocket = require('ws');
const ws = new WebSocket('ws://127.0.0.1:8080')
ws.on('open', function () {
for (var i = 0; i < 3; i++) {
ws.send('Hello from client: ' + i)
}
ws.on('message', function (msg) {
console.log(msg)
})
})
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
一个复杂一点的配置:
const WebSocket = require('ws');
const wss = new WebSocket.Server({
port: 8080,
perMessageDeflate: {
zlibDeflateOptions: {
// See zlib defaults.
chunkSize: 1024,
memLevel: 7,
level: 3
},
zlibInflateOptions: {
chunkSize: 10 * 1024
},
// Other options settable:
clientNoContextTakeover: true, // Defaults to negotiated value.
serverNoContextTakeover: true, // Defaults to negotiated value.
serverMaxWindowBits: 10, // Defaults to negotiated value.
// Below options specified as default values.
concurrencyLimit: 10, // Limits zlib concurrency for perf.
threshold: 1024 // Size (in bytes) below which messages
// should not be compressed.
}
});
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
# socket.io配置服务端
WebSocket是跟随HTML5一同提出的,所以在兼容性上存在问题,这时一个非常好用的库就登场了——Socket.io (opens new window)。
socket.io封装了websocket,同时包含了其它的连接方式,你在任何浏览器里都可以使用socket.io来建立异步的连接。socket.io包含了服务端和客户端的库,如果在浏览器中使用了socket.io的js,服务端也必须同样适用。
socket.io是基于 Websocket 的Client-Server 实时通信库。
socket.io底层是基于engine.io这个库。engine.io为 socket.io 提供跨浏览器/跨设备的双向通信的底层库。engine.io使用了 Websocket 和 XHR 方式封装了一套 socket 协议。在低版本的浏览器中,不支持Websocket,为了兼容使用长轮询(polling)替代。
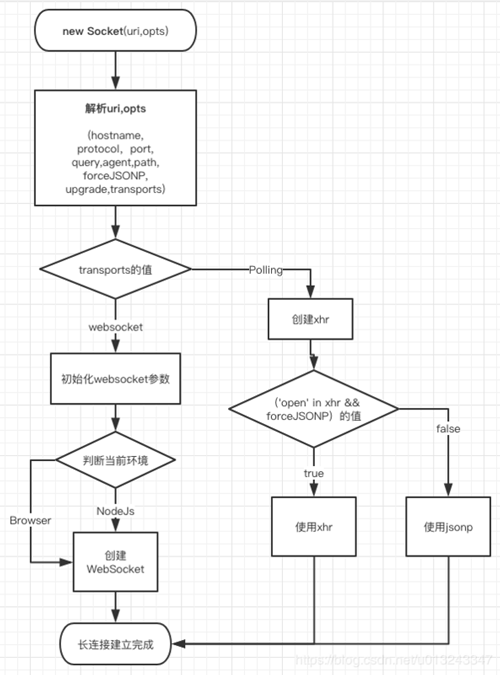
socket.io服务端 http / express两个库
const app = require('express')() const http = require('http').createServer(app) const io = require('socket.io')(http) app.get('/', function (req, res) { res.sendFile(__dirname + '/index.html') }) // 监听连接 io.on('connection', function (socket) { console.log('a socket is connected'); // 获取客户端的消息 socket.on('chat msg', function (msg) { console.log('msg from client: ' + msg); // 发送消息给客户端 socket.send('server says: ' + msg) }) }) http.listen(3000, function () { console.log('server is running on: 3000') })
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22客户端,需要引用
socket.io.js
,有两种方式去引用:(1)支持从socket.io.client中的dist中加载这个js (2)CDN<script src="https://cdn.jsdelivr.net/npm/socket.io-client@2/dist/socket.io.js"></script> <script> var socket = io(); </script>
1
2
3
4客户端的仓库地址:https://github.com/socketio/socket.io-client (opens new window)