# 7.Swagger ui
Swagger使用yaml文件去定义RESTful接口:
swagger: "2.0"
info:
version: "2.0.0"
title: "swagger test"
host: localhost:3000
basePath: /
schemes:
- http
consumes:
- application/json
produces:
- application/json
paths:
/get:
get:
tags:
- "test Swagger UI"
summary: "send get name"
description: "get request"
produces:
- "application/json"
parameters:
- name: "name"
in: "query"
description: "name of params"
required: true
type: "string"
responses:
200:
description: "successful operation"
schema:
$ref: "#/definitions/ApiResponse"
definitions:
ApiResponse:
type: "object"
properties:
code:
type: "integer"
format: "int32"
data:
type: "object"
message:
type: "string"
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
# Swagger Edit
Swagger Edit是Swagger接口管理的在线编辑器,可以实时去查看yaml对应生成的接口,以及接口测试。
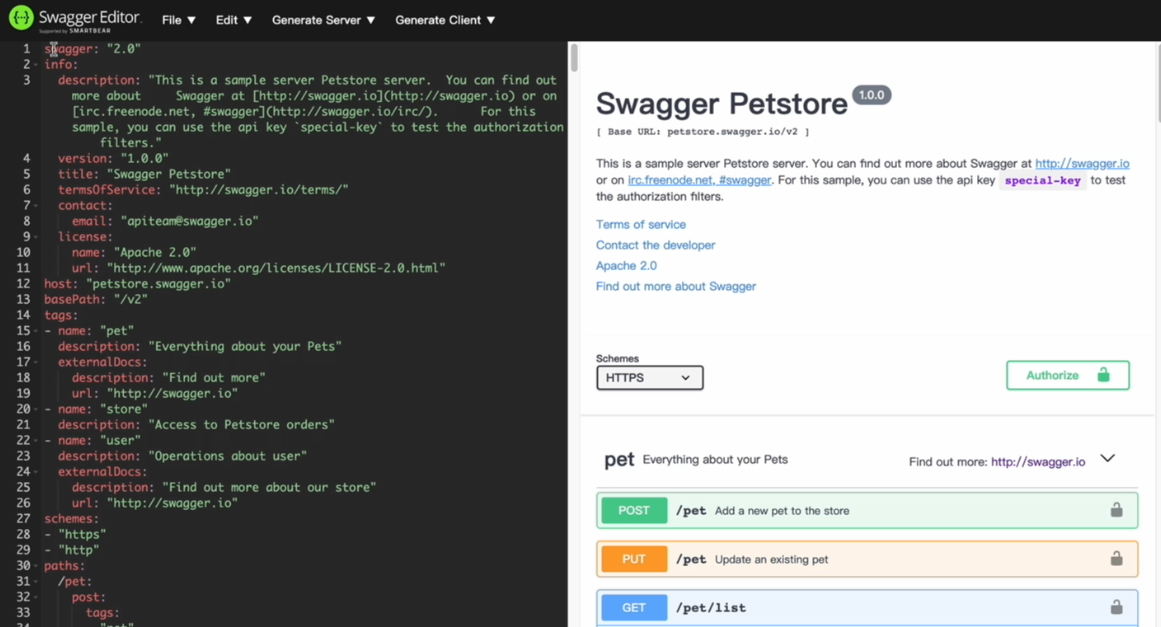
安装依赖
npm install -g swagger
1
可以在项目的根目录/api/swagger/swagger.yaml
去创建接口文件,使用swagger命令来进行编辑
swagger project edit
1
# Swagger UI
使用gulp去转化yaml的文件到json
使用全局安装命令来安装
gulp
npm install -g gulp-cli
1
安装依赖
npm install -D gulp js-yaml
1
创建gulpfile.js
const { watch } = require("gulp");
const yaml = require("js-yaml");
const path = require("path");
const fs = require("fs");
function defaultTask(cb) {
const doc = yaml.safeLoad(
fs.readFileSync(path.join(__dirname, "./api/swagger/swagger.yaml"))
);
fs.writeFileSync(
path.join(__dirname, "./public/swagger.json"),
JSON.stringify(doc, null, " ")
);
cb();
}
exports.default = function() {
watch("api/**/*.yaml", defaultTask);
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
使用gulp命令去转化yaml为json数据
- 使用vscode的live-server去实时的预览
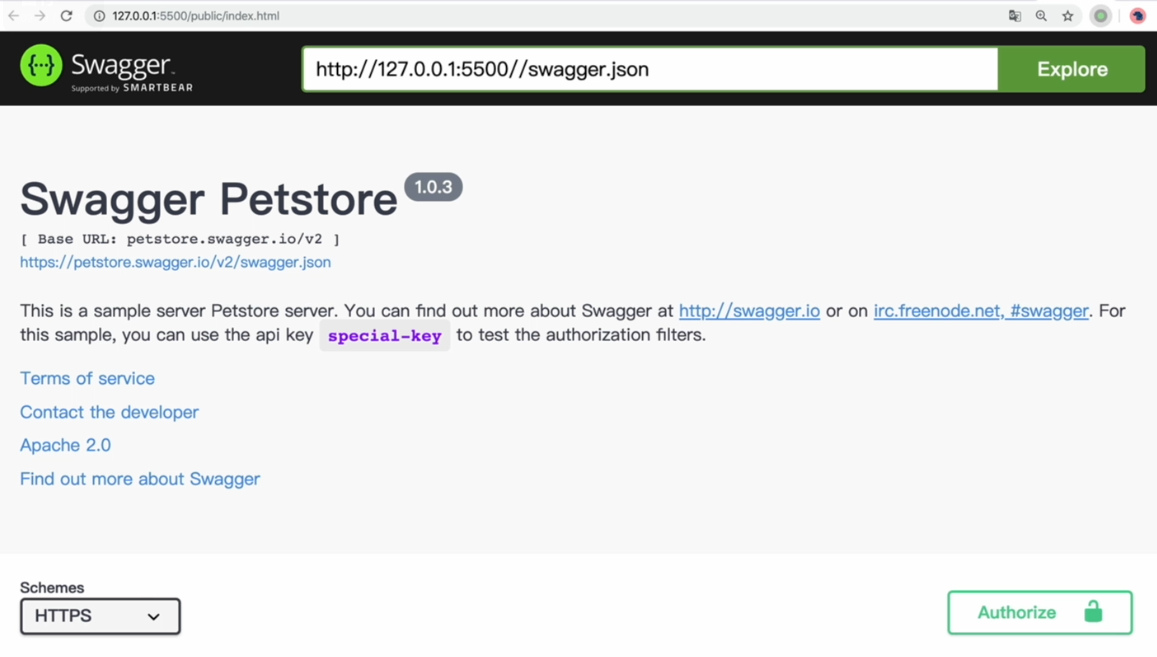
- 使用
koa-static
来进行自己管理
const Koa = require("koa");
const app = new Koa();
const Router = require("koa-router");
const jsonutil = require("koa-json");
const compose = require("koa-compose");
const koaBody = require("koa-body");
const cors = require("@koa/cors");
const serve = require("koa-static");
const path = require("path");
// compose 用于压缩中间件
const middleware = compose([
koaBody(),
cors(),
jsonutil({ pretty: false, param: "pretty" }),
serve(path.join(__dirname, "../public"))
]);
const router = new Router();
// get方法
router.get("/get", async ctx => {
const body = ctx.query;
ctx.body = {
data: body,
msg: `hello ${body.name}!`,
code: 200
};
});
// post方法
router.post("/post", async ctx => {
const { body } = ctx.request;
ctx.body = {
data: body,
msg: "hello world! Your name is " + body.name,
code: 200
};
});
// delete 方法
router.delete("/del", async ctx => {
ctx.body = { errmsg: "ok", errcode: 0 };
});
// put 方法
router.put("/put", async ctx => {
const { body } = ctx.request;
ctx.body = {
msg: "hello world! Your name is " + body.name,
code: 200
};
});
app.use(middleware);
app.use(router.routes());
app.listen(3000);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
就可以在localhost:3000
下来访问swagger ui了
← 6.Yapi(推荐) 8.ApiDoc →